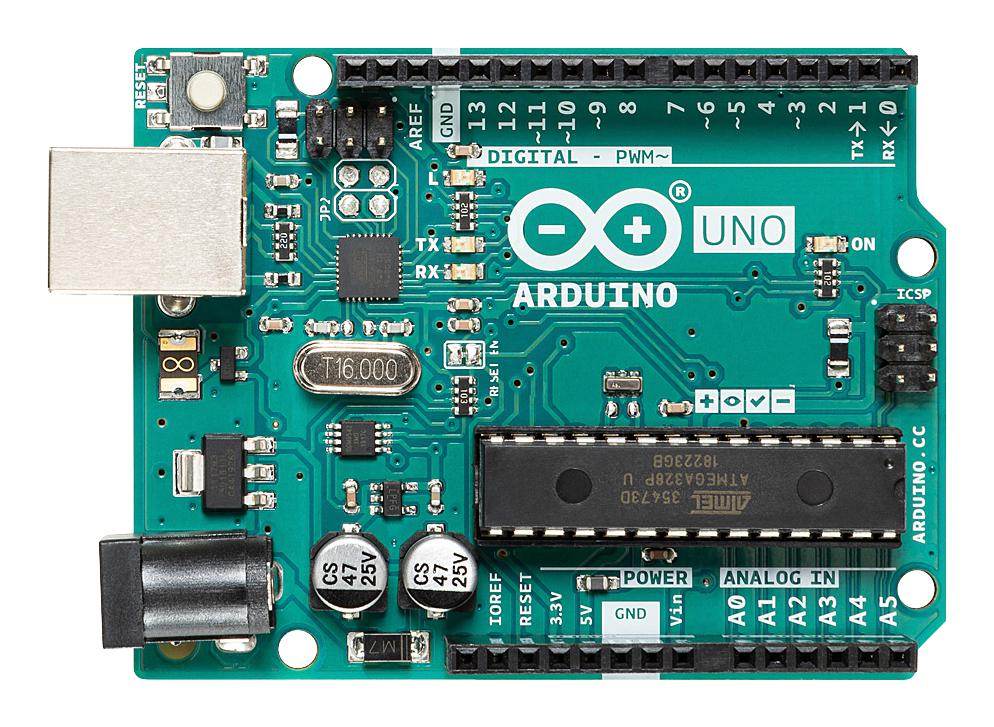
Arduino is the perfect board to get familiar with electronics and coding. Arduino is an open-source electronics platform based on easy-to-use hardware and software. Arduino boards can read inputs – light on a sensor, a finger on a button, or a Twitter message – and turn them into an output – activating a motor, turning on an LED, publishing something online. We can tell the Arduino board what to do by sending a set of instructions to the microcontroller on the board. To do so, we need to use the Arduino programming language (based on Wiring) and the Arduino Software (IDE), based on Processing. While all Arduino boards differ from each other, several key components can be found on practically any Arduino.
Components of an Arduino board
- Microcontroller – This is the brain of an Arduino, and is the component that we load programs into. Think of it as a tiny computer, designed to execute only a specific number of things.
- USB port – used to connect your Arduino board to a computer.
- USB to Serial chip – the USB to Serial is an important component, as it helps translate data that comes from e.g. a computer to the onboard microcontroller. This is what makes it possible to program the Arduino board from your computer.
- Digital pins – pins that use digital logic (0,1 or LOW/HIGH). Commonly used for switches and to turn on/off an LED.
- Analog pins – pins that can read analog values in a 10-bit resolution (0-1023).
- 5V / 3.3V pins- these pins are used to power external components.
- GND – also known as ground, negative or simply -, is used to complete a circuit, where the electrical level is at 0 volts.
- VIN – stands for Voltage In, where you can connect external power supplies.
Common Types of Arduino Boards
Arduino UNO
Arduino UNO is based on an ATmega328P microcontroller. The Arduino UNO includes 6 analog pin inputs, 14 digital pins, a USB connector, a power jack, and an ICSP (In-Circuit Serial Programming) header.
It is the most used and of standard form from the list of all available Arduino Boards. It is also recommended for beginners as it is easy to use.
Specifications
Microcontroller | ATmega328P – 8 bit AVR family microcontroller |
Operating Voltage | 5V |
Recommended Input Voltage | 7-12V |
Input Voltage Limits | 6-20V |
Analog Input Pins | 6 (A0 – A5) |
Digital I/O Pins | 14 (Out of which 6 provide PWM output) |
DC Current on I/O Pins | 40 mA |
DC Current on 3.3V Pin | 50 mA |
Flash Memory | 32 KB (0.5 KB is used for Bootloader) |
SRAM | 2 KB |
EEPROM | 1 KB |
Frequency (Clock Speed) | 16 MHz |
Arduino Nano
The Arduino Nano is a small Arduino board based on the ATmega328P or ATmega628 Microcontroller. The connectivity is the same as the Arduino UNO board.
The Nano board is defined as a sustainable, small, consistent, and flexible microcontroller board. It is small in size compared to the UNO board. The devices required to start our projects using the Arduino Nano board are Arduino IDE and mini USB.
The Arduino Nano includes an I/O pin set of 14 digital pins and 8 analog pins. It also includes 6 Power pins and 2 Reset pins.
Specifications
Microcontroller | ATmega328P – 8-bit AVR family microcontroller |
Operating Voltage | 5V |
Recommended Input Voltage for Vin pin | 7-12V |
Analog Input Pins | 6 (A0 – A5) |
Digital I/O Pins | 14 (Out of which 6 provide PWM output) |
DC Current on I/O Pins | 40 mA |
DC Current on 3.3V Pin | 50 mA |
Flash Memory | 32 KB (2 KB is used for Bootloader) |
SRAM | 2 KB |
EEPROM | 1 KB |
Frequency (Clock Speed) | 16 MHz |
Communication | IIC, SPI, USART |
Arduino Mega
The Arduino Mega is based on the ATmega2560 Microcontroller. The ATmega2560 is an 8-bit microcontroller. We need a simple USB cable to connect to the computer and the AC to DC adapter or battery to get started with it. It has the advantage of working with more memory space.
The Arduino Mega includes 54 I/O digital pins and 16 Analog Input/Output (I/O), ICSP header, a reset button, 4 UART (Universal Asynchronous Receiver/Transmitter) ports, USB connection, and a power jack.
Specifications
Microcontroller | ATmega2650 |
Operating Voltage | 5V |
Recommended Input Voltage for Vin pin | 7-12V |
Analog Input Pins | 16 |
Digital I/O Pins | 54 (Out of which 6 provide PWM output) |
DC Current on I/O Pins | 40 mA |
DC Current on 3.3V Pin | 50 mA |
Flash Memory | 256 KB (8 KB is used for Bootloader) |
SRAM | 8 KB |
EEPROM | 4 KB |
Frequency (Clock Speed) | 16 MHz |
How to use
The Arduino Uno is programmed using the Arduino Software (IDE), an Integrated Development Environment common to all boards and running both online and offline. If you want to program your Arduino while offline, you need to install the Arduino Desktop IDE. You must have installed the Arduino Software (IDE) on your PC. Connect your Uno board with an A B USB cable; sometimes, this cable is called a USB printer cable.
The USB connection with the PC is necessary to program the board and not just to power it up. The Uno automatically draws power from either the USB or an external power supply. Connect the board to your computer using the USB cable. The green power LED (labelled PWR) should go on.
Install the board drivers
If you used the Installer, Windows – from XP up to 11 – would install drivers automatically as soon as you connect your board.
If you had downloaded and expanded the Zip package or, for some reason, the board wasn’t properly recognized, please follow the procedure below.
- Click on the Start Menu, and open up the Control Panel.
- While in the Control Panel, navigate to System and Security. Next, click on System. Once the System window is up, open the Device Manager.
- Look under Ports (COM & LPT). You should see an open port named “Arduino UNO (COMxx)”. If there is no COM & LPT section, look under “Other Devices” for “Unknown Device”.
- Right click on the “Arduino UNO (COmxx)” port and choose the “Update Driver Software” option.
- Next, choose the “Browse my computer for driver software” option.
- Finally, navigate to and select the driver file named “arduino.inf”, located in the “Drivers” folder of the Arduino Software download (not the “FTDI USB Drivers” sub-directory). If you are using an old version of the IDE (1.0.3 or older), choose the Uno driver file named “Arduino UNO.inf”
- Windows will finish up the driver installation from there.
Open your first sketch
Open the LED blink example sketch: File > Examples >01.Basics > Blink.
Select your board type and port
You’ll need to select the entry in the Tools > Board menu that corresponds to your Arduino board.
Select the serial device of the board from the Tools | Serial Port menu. This is likely to be COM3 or higher (COM1 and COM2 are usually reserved for hardware serial ports). To find out, you can disconnect your board and re-open the menu; the entry that disappears should be the Arduino board. Reconnect the board and select that serial port.
Upload the program
Now, add the code – https://www.arduino.cc/en/Tutorial/BuiltInExamples/Blink & simply click the “Upload” button in the environment. Wait a few seconds – you should see the RX and TX LEDs on the board flashing. If the upload is successful, the message “Done uploading.” will appear in the status bar.
A few seconds after the upload finishes, you should see the pin 13 (L) LED on the board start to blink (in orange). If it does, congratulations! You’ve gotten Arduino up and running.
Common Applications
Arduinos are excellent for beginner and intermediate robotics projects. They are powerful enough to be able to give the basic commands the robot needs to function while keeping their limited resources focused on the tasks at hand. This eliminates interference or resource drain from other programs running in the background. Teachers and students use it to build low-cost scientific instruments, to prove chemistry and physics principles, or to get started with programming and robotics. Designers and architects build interactive prototypes, and musicians and artists use it for installations and to experiment with new musical instruments.
Some example projects that students can try out in the ATL Lab
1. Blinking an LED – https://docs.arduino.cc/built-in-examples/basics/Blink
2. Fading an LED – https://docs.arduino.cc/built-in-examples/basics/Fade
3. Analog Read Serial – https://docs.arduino.cc/built-in-examples/basics/AnalogReadSerial
4. Analog In, Out Serial – https://docs.arduino.cc/built-in-examples/analog/AnalogInOutSerial
Important Links
Learn the basics of Electronics & Arduino – Learn | Arduino Documentation | Arduino Documentation (https://docs.arduino.cc/learn/)
How to install & use the Arduino IDE – Arduino IDE 2 Tutorials | Arduino Documentation | Arduino Documentation (https://docs.arduino.cc/software/ide-v2)
Arduino Built in Examples – Built-in Examples | Arduino Documentation | Arduino Documentation (https://docs.arduino.cc/built-in-examples/)
How to use the Arduino Cloud – Arduino Cloud | Arduino Documentation | Arduino Documentation (https://docs.arduino.cc/arduino-cloud/)
Understanding the Arduino Programming Language – https://www.arduino.cc/reference/en/ (https://www.arduino.cc/reference/en/)
Exciting Projects that can be built using Arduino – Arduino Project Hub (https://create.arduino.cc/projecthub)